Wow! I thought it would be MUCH easier to find the answer to this. Because I had so much trouble, I’m creating this post. With any luck, someone else will find it when they go looking.
The Problem
I created a login form in Angular 10 and wanted the focus to be on the first input when the page rendered.
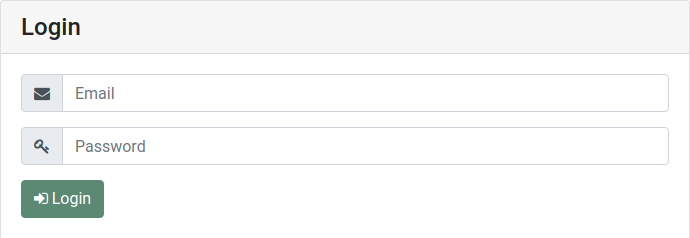
HTML’s ‘autofocus’ did not work. I found lots of Directives and references to using Renderer (from early Angular it seems). However, nothing worked for me.
The Solution
I used a bunch of resources to bring together a solution that works and is, I believe, safe to use.
Typescript
import { Component, OnInit, ViewChild, AfterViewInit, ElementRef } from '@angular/core'; @Component({ selector: 'app-login', templateUrl: './login.component.html' }) export class LoginComponent implements OnInit, AfterViewInit { @ViewChild('email') email: ElementRef; constructor() { } ngAfterViewInit(): void { this.email.nativeElement.focus(); } }
In the component, import ViewChild, ElementRef, and AfterViewInit from ‘@angular/core’. Make sure the class implements the AfterViewInit interface. Add a class member, in this case called ’email’ of type ElementRef with a @ViewChild decorator. The value in the parenthesis of the decorator is the Angular ID (see HTML below).
Then, create a method called ngAfterViewInit. This is where the magic actually happens as this method is called once the view has been initialized. In ngAfterViewInit, we will set the focus to the desired element.
HTML
<input #email type="email" name="email">
In the HTML, add the angular ID (not what it’s called, but looks like a CSS ID selector and Angular recognizes it), the string that was in the ViewChild decorator, but here with a ‘#’ in front, ‘#email.
Summary
That should be it. It is super easy, functional, and I believe it is safe. There are some issues with accessing DOM elements directly, but my understanding is that using the ElementRef is a safe method of doing so. If I have left anything out or this is unclear, let me know.